Are you gearing up for a C/C++ interview and looking to brush up on some frequently asked top C++ interview questions? Look no further! Here at Codes Navigator we have a detailed list of the top FAQs encountered in C/C++ interviews. Whether you’re a beginner or a experienced developer, these questions cover a wide range of topics and can serve as valuable preparation for your upcoming interview. In this article we have combined all the important questions asked in top MNC’s like TCS, Cognizant, Accenture, Capgemini, Infosys, Wipro, Tech Mahindra, HCL, etc.
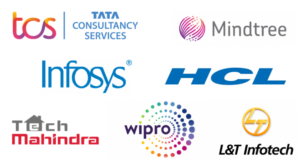
Top C/C++ interview questions
What is the syntax to declare a variable in C?
In C, you declare a variable by specifying its data type followed by the variable name. For example:
// Declares an integer variable named myVariable
int myVariable;
// Example
int value;
You can also initialize the variable at the time of declaration:
// Declares an integer variable named value and initializes it with the value 10
int value = 10;
Explain the difference between ‘int’ and ‘float’ data types in C/C++.
In C, ‘int’ and ‘float’ are different data types used to represent numerical values, but they have distinct characteristics:
Integer (int):
- Integers are whole numbers without any fractional or decimal part.
- They can be positive, negative, or zero.
- The ‘int’ data type typically occupies a fixed amount of memory, commonly 2 bytes on most modern systems.
- It is suitable for representing quantities that are counted or indexed, such as the number of elements in an array or loop counters.
- Example:
int myInteger = 10;
Floating Point (float):
- Floating-point numbers are real numbers that can have fractional parts.
- They can represent a wider range of values compared to integers, including very small or very large numbers.
- The ‘float’ data type typically occupies 4 bytes of memory.
- Floating-point numbers are useful for representing quantities that require precision, such as measurements or scientific computations.
- Example:
float myFloat = 3.14;
In summary, the main difference between ‘int’ and ‘float’ in C is that ‘int’ represents integers (whole numbers), while ‘float’ represents floating-point numbers (real numbers with fractional parts). The choice between them depends on the nature of the data you need to work with and the level of precision required in your calculations.
Explain the difference between ‘=’ and ‘==’ operators in C/C++.
In C, the ‘=’ operator and the ‘==’ operator used for different purposes:
‘=’ Operator (Assignment Operator):
- The ‘=’ operator is used for assignment in C.
- It assigns the value on the right-hand side of the operator to the variable on the left-hand side.
- Example:
int x = 10;
assigns the value 10 to the variable ‘x’.
‘==’ Operator (Equality Operator):
- The ‘==’ operator is used for comparison in C.
- It checks whether the values on both sides of the operator are equal.
- If the values are equal, the expression evaluates to true (1); otherwise, it evaluates to false (0).
- Example:
if (x == 10)
checks if the value of ‘x’ is equal to 10.
Explain the difference between ‘while’, ‘do-while’ and ‘for’ loops.
In C/C++, ‘while’, ‘do-while’, and ‘for’ loops are all used for repetitive execution of code, but they have different structures and behaviors:
‘while’ Loop:
- The ‘while’ loop is a pre-test loop, meaning the condition is tested before entering the loop body.
- It executes the loop body as long as the condition is true.
- Example:
while (condition) {
//
// Loop body
//
}
‘do-while’ Loop:
- The ‘do-while’ loop is a post-test loop, meaning the condition is tested after executing the loop body at least once.
- It executes the loop body first and then checks the condition.
- It guarantees that the loop body will execute at least once.
- Example:
do {
//
// Loop body
//
} while (condition);
‘for’ Loop:
- The ‘for’ loop provides a compact way to express loops with initialization, condition, and increment/decrement all in one line.
- It is often used when the number of iterations is known beforehand.
- Example:
for (initialization; condition; increment/decrement) {
//
// Loop body
//
}
In summary, ‘while’ and ‘do-while’ loops are used when the number of iterations is not known beforehand, while the ‘for’ loop is typically used when the number of iterations is known or when a loop counter is needed. Each type of loop has its own use cases and advantages, so choosing the appropriate loop structure depends on the specific requirements of the task at hand.
What is the purpose of the ‘switch’ statement?
The ‘switch’ statement in C/C++ provides a way to execute different blocks of code based on the value of a single expression or variable. Its primary purpose is to streamline decision-making in situations where multiple branches of code need to be executed depending on the value of a single variable.
Key features of the ‘switch’ statement:
- Expression Evaluation: The ‘switch’ statement evaluates an expression and compares its value against a series of constant integral expressions (case labels).
- Case Labels: Each ‘case’ label specifies a constant value that the expression is compared against. If the expression matches a case label, the corresponding block of code is executed.
- Default Case: The ‘default’ case is optional and is executed when none of the ‘case’ labels match the value of the expression.
- Break Statement: The ‘break’ statement is used to exit the switch statement. It prevents execution from falling through to subsequent cases.
Here’s a basic example of a ‘switch’ statement:
switch (expression) {
case constant1:
// Code to execute if expression equals constant1
break;
case constant2:
// Code to execute if expression equals constant2
break;
// More cases can be added as needed
default:
// Code to execute if expression doesn't match any case
}
In summary, the ‘switch’ statement is used in C to simplify decision-making by selecting among multiple code blocks based on the value of an expression.
What is a pointer in C? How do you declare and initialize it?
In C, a pointer is a variable that stores the memory address of another variable. Pointers allow indirect access to variables, enabling dynamic memory allocation, efficient array manipulation, and passing parameters by reference in functions.
To declare a pointer in C, you use the asterisk (*) symbol followed by the data type of the variable it points to. Here’s the syntax:
data_type *pointer_name;
For example, to declare a pointer to an integer variable:
int *ptr;
In both cases, ptr now “points to” the memory location of the variable num. This allows you to indirectly access and manipulate the value of num through the pointer ptr. It’s important to note that uninitialized pointers contain garbage values, so it’s best practice to initialize pointers before using them.
What is the purpose of the ‘NULL’ pointer in C?
In C, the ‘NULL’ pointer serves as a special value that indicates that the pointer does not point to any valid memory location. It’s essentially a reserved keyword representing a pointer that does not reference any object.
The purpose of the ‘NULL’ pointer is to provide a standard way to represent an uninitialized or invalid pointer, allowing for more robust error handling and memory management. When a pointer is assigned the value ‘NULL’, it signifies that it is not pointing to any meaningful memory address. This can be particularly useful when dealing with dynamic memory allocation, function returns, and error conditions.
Here’s a common usage example:
int *ptr = NULL; // Initialize ptr to NULL
// Code that might assign a valid memory address to ptr based on certain conditions
if (ptr == NULL) {
// Handle error condition where ptr is not initialized or valid
}
In this example, if the pointer ‘ptr’ has not been assigned a valid memory address, it will be initialized to ‘NULL’. Later, the program can check whether ‘ptr’ is still ‘NULL’ to determine if an error condition occurred or if the pointer needs to be initialized.
Using ‘NULL’ helps to prevent undefined behavior and segmentation faults that can occur when dereferencing uninitialized or invalid pointers. It’s a convention in C programming to use ‘NULL’ to represent pointers that do not point to valid memory locations, enhancing the safety and reliability of C programs.
How do you allocate memory dynamically using pointers in C?
In C, you can allocate memory dynamically at runtime using pointers and specific functions like malloc
, calloc
, or realloc
. Here’s a brief explanation of each method:
- malloc: Stands for “memory allocation.” It allocates a block of memory of a specified size in bytes and returns a pointer to the beginning of that block. The memory allocated by malloc is uninitialized, meaning it contains garbage values.
int *ptr = (int *)malloc(5 * sizeof(int)); // Allocates memory for an array of 5 integers
- calloc: Stands for “contiguous allocation.” It allocates memory for an array of elements, each of a specified size, and initializes all bits to zero. This function is useful when you want to ensure that the allocated memory is initialized.
int *ptr = (int *)calloc(5, sizeof(int)); // Allocates memory for an array of 5 integers and initializes them to zero
- realloc: Stands for “re-allocation.” It changes the size of the previously allocated memory block. If the size is increased, the additional memory is uninitialized. If the size is decreased, the excess memory is deallocated.
int *ptr = (int *)malloc(5 * sizeof(int)); // Allocates memory for an array of 5 integers
ptr = (int *)realloc(ptr, 10 * sizeof(int)); // Resizes the allocated memory block to hold 10 integers
After dynamically allocating memory, you can use the allocated memory through the pointer returned by these functions. Remember to free the dynamically allocated memory using the free
function when it’s no longer needed to prevent memory leaks.
free(ptr); // Frees the dynamically allocated memory
Dynamic memory allocation using pointers in C is essential for scenarios where the size of data structures is not known at compile time or when memory needs to be allocated and deallocated dynamically during program execution.
- Cognizant GenC Top 25+ Technical Interview Questions
- Cognizant GenC Pro Top 25+ Technical Interview Questions (2025)
- Cognizant GenC Next Top 30+ Technical Interview Questions
- Cognizant Communication Assessment 2025 Batch
- TCS Digital Interview Experience #1
Explain the difference between pass by value and pass by reference using pointers.
In C, pass by value and pass by reference are two different mechanisms for passing arguments to functions, and pointers play a crucial role in distinguishing between them:
- Pass by Value:
- In pass by value, a copy of the argument’s value is passed to the function.
- The function works with a local copy of the argument, so any modifications made to the parameter inside the function do not affect the original variable outside the function.
- This method is efficient for simple data types and is safer because it prevents unintended changes to the original data.
- Example:
void increment(int x) {
x++; // Increment local copy of x
}
int main() {
int num = 10;
increment(num); // Pass num by value
// num remains unchanged because it was passed by value
}
- Pass by Reference using Pointers:
- In pass by reference, instead of passing a copy of the argument’s value, the function receives the memory address (reference) of the argument using a pointer.
- Any modifications made to the parameter inside the function directly affect the original variable outside the function because both the function parameter and the original variable refer to the same memory location.
- Pass by reference is useful when you need a function to modify the original variable or when dealing with large data structures to avoid the overhead of copying.
- Example:
void incrementByReference(int *ptr) {
(*ptr)++; // Increment the value pointed to by ptr
}
int main() {
int num = 10;
incrementByReference(&num); // Pass num by reference using a pointer
// num is now 11 because it was modified by the function
}
In summary, pass by value involves passing a copy of the argument’s value, while pass by reference using pointers involves passing the memory address of the argument. Pass by value is safer but less efficient for large data, while pass by reference allows for direct modification of the original data but requires careful handling to avoid unintended side effects.
How do you free dynamically allocated memory in C?
In C, dynamically allocated memory should be manually deallocated to prevent memory leaks and ensure efficient memory usage. The ‘free()‘ function is used to release dynamically allocated memory. Here’s how you can free dynamically allocated memory in C:
- Allocate memory using ‘
malloc()', 'calloc()', or 'realloc()'
:
int *ptr = (int *)malloc(sizeof(int) * size);
or
int *ptr = (int *)calloc(size, sizeof(int));
or
int *ptr = (int *)realloc(NULL, sizeof(int) * size);
- Use the allocated memory as needed.
- Free the allocated memory using the ‘
free()
‘ function when it’s no longer needed:
free(ptr);
It’s crucial to remember a few key points when using ‘free()’ to deallocate memory:
- Only dynamically allocated memory should be freed. Attempting to free statically allocated memory or memory that has already been freed can lead to undefined behavior.
- Always free dynamically allocated memory before losing access to the pointer that points to it. Failing to do so can result in memory leaks.
- After calling ‘free()’, the pointer becomes a dangling pointer, meaning it points to memory that has been deallocated. Avoid dereferencing or using dangling pointers to prevent undefined behavior.
By following these guidelines and properly managing dynamically allocated memory with ‘free()’, you can ensure efficient memory usage and avoid memory-related issues in C programs.
What is the difference between “++i” and “i++”?
In C, both “++i” and “i++” are unary increment operators used to increment the value of a variable ‘i’ by 1. However, there is a subtle difference between them in terms of when the increment operation occurs and the value they return:
- “++i” (Pre-increment):
- “++i” is a pre-increment operator.
- It first increments the value of ‘i’ and then returns the incremented value.
- The increment operation occurs before the value of ‘i’ is used in any other expression.
- Example:
int i = 5;
int result = ++i; // Increment i by 1, then assign the incremented value to result
// Now, i = 6 and result = 6
- “i++” (Post-increment):
- “i++” is a post-increment operator.
- It first uses the current value of ‘i’ in the expression where it appears and then increments ‘i’.
- The increment operation occurs after the value of ‘i’ is used in any other expression.
- Example:
int i = 5;
int result = i++; // Assign the current value of i to result, then increment i by 1
// Now, i = 6 and result = 5
Explain the purpose of the “typedef” keyword in C.
In C, the “typedef” keyword is used to create an alias or alternative name for existing data types. It allows programmers to define custom names for complex or frequently used data types, improving code readability, portability, and maintainability.
The primary purpose of “typedef” is to enhance the clarity and abstraction of code by providing descriptive names for data types, especially when dealing with complex structures or function pointers. It also simplifies code modifications by enabling easy changes to data types throughout the codebase.
Here’s an example demonstrating the use of “typedef” to create an alias for a data type:
typedef struct {
int x;
int y;
} Point;
// Now, "Point" can be used as an alias for "struct { int x; int y; }"
int main() {
Point p1 = {10, 20};
// Instead of "struct { int x; int y; } p1 = {10, 20};", we can use "Point p1 = {10, 20};"
return 0;
}
In this example, “typedef” creates an alias “Point” for the structure containing integer fields ‘x’ and ‘y’. This allows us to declare variables of type “Point” instead of using the more cumbersome “struct { int x; int y; }”.
Overall, the “typedef” keyword in C enhances code clarity, abstraction, and maintainability by providing custom names for data types, making the code more expressive and easier to understand.
Explain the difference between local and global variables in C.
In C, local and global variables are two types of variables with different scopes and lifetimes:
- Local Variables:
- Local variables are declared within a function or block and can only be accessed within that function or block.
- They are created when the function or block is entered and destroyed when the function or block exits.
- Local variables are typically used for storing temporary data or intermediate results within a specific function or block.
- They are not accessible from outside the function or block in which they are declared.
- Example:
void myFunction() {
int localVar = 10; // localVar is a local variable
}
- Global Variables:
- Global variables are declared outside of any function or block and can be accessed from any part of the program.
- They are created when the program starts and destroyed when the program terminates.
- Global variables have a lifetime that spans the entire execution of the program.
- They are typically used for storing data that needs to be shared among multiple functions or modules.
- Global variables can be accessed and modified from any part of the program, which can lead to unintended side effects if not managed carefully.
- Example:
int globalVar = 10; // globalVar is a global variable
void myFunction() {
// globalVar can be accessed and modified here
}
Explain the concept of recursion in C with an example..
Recursion is a programming technique in which a function calls itself directly or indirectly to solve a problem. In C, recursive functions offer an elegant solution for solving problems that can be broken down into smaller, similar sub-problems.
Here’s an example of a recursive function to calculate the factorial of a non-negative integer:
#include <stdio.h>
// Recursive function to calculate the factorial of a non-negative integer
unsigned long long factorial(int n) {
// Base case: factorial of 0 is 1
if (n == 0) {
return 1;
}
// Recursive case: calculate factorial of (n-1) and multiply by n
else {
return n * factorial(n - 1);
}
}
int main() {
int num = 5;
unsigned long long fact = factorial(num);
printf("Factorial of %d is %llu\n", num, fact);
return 0;
}
Explanation:
- The
factorial()
function takes an integern
as input and returns its factorial. - In the base case (when
n
equals 0), the function returns 1 because the factorial of 0 is defined to be 1. - In the recursive case, the function calls itself with
n-1
and multiplies the result byn
. This process continues until the base case is reached. - The
main()
function demonstrates how to use thefactorial()
function by calculating and printing the factorial of 5.
How do you create and use arrays of structures in C?
In C, you can create and use arrays of structures by defining a structure and then declaring an array of that structure type. Here’s a step-by-step guide with an example:
- Define the structure:
First, define the structure that represents the data you want to store. Each element of the array will be an instance of this structure.
- Declare and initialize the array:
Declare an array of structures by specifying the structure type followed by the array name and size.
- Accessing array elements:
You can access individual elements of the array using the array index notation ([]
).
- Iterating through the array:
You can iterate through the array using loops, such asfor
orwhile
, to access or modify each element.
Putting it all together:
#include <stdio.h>
#include <string.h>
struct Student {
int id;
char name[50];
float marks;
};
int main() {
struct Student students[5]; // Array of 5 Student structures
// Initialize array elements
students[0].id = 1;
strcpy(students[0].name, "John");
students[0].marks = 85.5;
students[1].id = 2;
strcpy(students[1].name, "Alice");
students[1].marks = 90.0;
// Iterate through the array and print details
for (int i = 0; i < 2; i++) {
printf("Student ID: %d\n", students[i].id);
printf("Name: %s\n", students[i].name);
printf("Marks: %.2f\n", students[i].marks);
}
return 0;
}
This program declares an array of Student
structures, initializes some elements, and then iterates through the array to print the details of each student.
- Cognizant GenC Top 25+ Technical Interview Questions
- Cognizant GenC Pro Top 25+ Technical Interview Questions (2025)
- Cognizant GenC Next Top 30+ Technical Interview Questions
- Cognizant Communication Assessment 2025 Batch
- TCS Digital Interview Experience #1
How do you implement dynamic memory allocation using “malloc()” and “free()” functions?
Dynamic memory allocation in C using the malloc()
and free()
functions allows you to allocate and deallocate memory dynamically during program execution. Here’s how you can implement dynamic memory allocation:
- Allocate Memory using
malloc()
:
- Use the
malloc()
function to allocate memory dynamically. - Syntax:
void *malloc(size_t size);
- It returns a pointer to the allocated memory block or
NULL
if the allocation fails. - Example:
int *ptr = (int *)malloc(sizeof(int) * 5); // Allocate memory for 5 integers
if (ptr == NULL) {
printf("Memory allocation failed\n");
exit(1);
}
- Use the Allocated Memory:
- Once memory is allocated, you can use it just like any other memory.
- Example:
for (int i = 0; i < 5; i++) {
ptr[i] = i + 1; // Assign values to the allocated memory
}
- Deallocate Memory using
free()
:
- After you are done using the dynamically allocated memory, you should free it using the
free()
function to prevent memory leaks. - Syntax:
void free(void *ptr);
- It deallocates the memory block pointed to by
ptr
, releasing the memory back to the system. - Example:
free(ptr); // Free the dynamically allocated memory
By following these steps, you can effectively allocate and deallocate memory dynamically in C, ensuring efficient memory usage and preventing memory leaks.
What are the differences between arrays and pointers in C?
In C, arrays and pointers are related concepts but have important differences in their behavior and usage:
- Memory Allocation:
- Arrays: In C, arrays are a fixed-size contiguous block of memory where elements are stored sequentially. The size of the array must be specified at compile time.
- Pointers: Pointers, on the other hand, are variables that store memory addresses. They can point to dynamically allocated memory or existing data in memory.
- Size Information:
- Arrays: The size of an array is fixed and determined at compile time. The size of the array is implicitly known to the compiler.
- Pointers: Pointers do not inherently contain size information. They only hold the memory address of the data they point to. To determine the size of dynamically allocated memory, you typically need to track it separately.
- Modification:
- Arrays: The elements of an array are fixed in size and location. Once an array is declared, its size cannot be changed, and elements cannot be added or removed.
- Pointers: Pointers can be reassigned to point to different memory locations. They can also be used to iterate through arrays or dynamically allocated memory, allowing for more flexibility in data manipulation.
- Syntax:
- Arrays: Array elements are accessed using the array index notation (
[]
). Arrays are accessed directly by their name. - Pointers: Pointers are dereferenced using the indirection operator (
*
). Pointers must be dereferenced to access the data they point to.
- Initialization:
- Arrays: Arrays can be initialized using brace-enclosed lists of values. The size of the array is determined by the number of elements provided during initialization.
- Pointers: Pointers can be initialized with memory addresses using the address-of operator (
&
), or they can be assigned the value of another pointer.
- Passing to Functions:
- Arrays: When passed to functions, arrays decay into pointers to their first elements. This means that the function receives a pointer to the array’s first element, not a copy of the entire array.
- Pointers: Pointers can be passed to functions directly. Changes made to the pointed-to data inside the function are reflected outside the function.
What is the purpose of the “void” pointer in C? When is it used?
The “void” pointer in C is a special type of pointer that is not associated with any specific data type. It is a generic pointer that can hold the address of any data type, including fundamental types (int, float, etc.), structures, and even functions.
The purpose of the “void” pointer is to provide a flexible mechanism for handling memory addresses without specifying the data type. This allows for greater versatility in programming, particularly in scenarios where the data type is unknown or when dealing with functions that can operate on different data types.
Here are some common use cases for “void” pointers in C:
- Memory Allocation
- Generic Functions
- Callback Functions
- Interfacing with External Libraries
- Memory Manipulation
Despite their flexibility, it’s important to handle “void” pointers with care, as they lack type safety. Improper use of “void” pointers can lead to undefined behavior, memory corruption, or segmentation faults. Typecasting should be done cautiously and only when necessary to avoid unintended consequences.
How do you implement a linked list using pointers in C?
Read full answer here
0 Comments